ManyTag Colour eInk Badge SDK - Minimum Viable Example for Android
Last year, I reviewed a Four-Colour eInk Name Badge - the ManyTag HSN371. The hardware itself is perfectly fine, but the Android app isn't great. It is complicated, crash-prone, and not available in the app-store.
After some back-and-forth with the manufacturer, they agreed to send me their Android SDK and documentation. Sadly, the PDF they sent me was riddled with errors and the software library is also a bit dodgy. So, with the help of Edward Toroshchyn and a hefty amount of automated boiler-plate, I managed to get it working.
The full code is open source, but here's a quick walk-through of the important bits.
First, the AAR library needs to be imported into the project. Place it in app/libs
and then include it in the Gradle build file:
JAVA
dependencies { implementation(files("libs/badge_nfc_api-release.aar")) }
The key to getting it working is in the Android permissions. It needs Bluetooth, NFC, and location. So the manifest has to contain:
XML<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
<uses-permission android:name="android.permission.BLUETOOTH_SCAN"/>
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT"/>
<uses-permission android:name="android.permission.NFC"/>
<uses-permission android:name="android.permission.NFC_TRANSACTION_EVENT"/>
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
The following imports are needed from the Android Archive library:
JAVA
import cn.manytag.badge_nfc_api.manager.BadgeWriteManager import cn.manytag.badge_nfc_api.manager.OnNFCReaderCallback import cn.manytag.badge_nfc_api.manager.OnBluetoothCallBack import cn.manytag.badge_nfc_api.manager.OnSendImageCallback
The library needs to be initialised with:
JAVA
val state = BadgeWriteManager.getInstance().init(this)
When the phone reads the NFC tag, it gets a bunch of information:
JAVA
BadgeWriteManager.getInstance().setOnNFCReaderCallback(object : OnNFCReaderCallback { override fun onReaderMessage(i: Int, tag: Tag) { if (i == 0) { BadgeWriteManager.getInstance().readNFC(tag) } } // Get the data from the badge override fun onReaderType(tag: Tag, isodep: IsoDep, i: Int, type: String, size: String, color: String) { if (i == 0) { nfcData = """ NFC Tag Detected!!! Tag: $tag IsoDep: $isodep Type: $type Size: $size Color: $color """.trimIndent() colorFromNFC = color tagObject = tag isoDepObject = isodep badgeType = type badgeSize = size } } })
The color
is most important right now. It says whether the badge is black and white, or black and white and red, or black and white and red and yellow.
After picking an image from the filesystem, it needs to be dithered into the correct colour format:
JAVA
processedBitmap = originalBitmap?.let { bitmap -> colorFromNFC?.let { color -> BadgeWriteManager.getInstance().processImage(bitmap, color) } }
Finally, the processed image needs to be converted to bytes and then sent to the badge via Bluetooth:
JAVA
if (processedBitmap != null && badgeType != null && badgeSize != null && colorFromNFC != null) { val imgData = BadgeWriteManager.getInstance().getImageData(processedBitmap!!, colorFromNFC!!) BadgeWriteManager.getInstance().sentImageResource( tagObject!!, isoDepObject!!, imgData, badgeType!!, badgeSize!!, colorFromNFC!! ) }
I realise this is a bit "draw the rest of the owl" but that should be enough to get you started on building an app which can communicate with these badges.
The app I've built isn't the prettiest in the world but at least it works. It scans a badge, gets its info, picks an image, dithers it, then sends it to the badge.
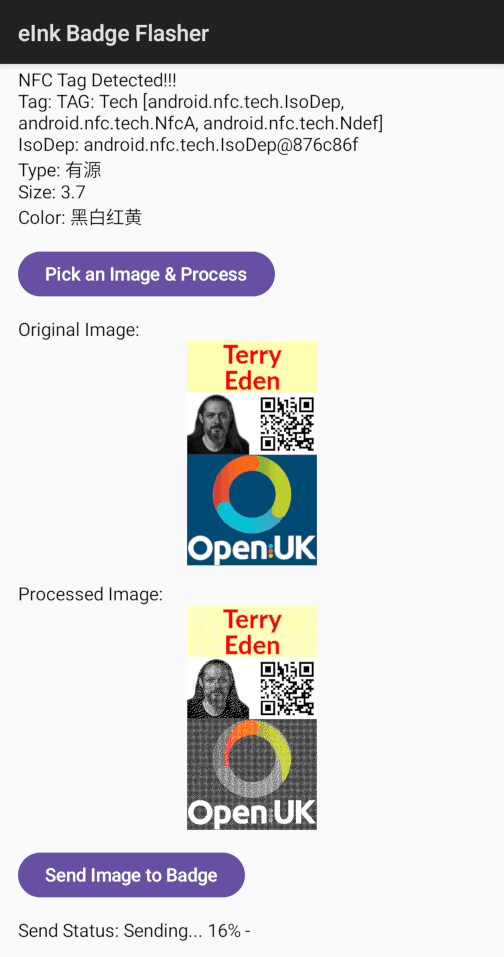
You can play with the code on CodeBerg.