Easy Tutorial For Getting Twitter Friends Using Python & Tweepy
Here's a very simple introduction to getting started with Tweepy - a Python program which lets you access Twitter. This will work on small computers like the Raspberry Pi.
Everything here takes place in the Terminal on the Command Line. This should work on Windows and Mac - but I'm using Linux.
Get Python
Open your terminal or command prompt. Type python
You should see something like:
Python 2.7.9 (default, Dec 28 2016, 18:26:44)
[GCC 4.8.4] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>>
If you do, you have Python installed! Press [ctrl]
and d
to exit.
If you get an error, you will need to install Python
Install Tweepy
Tweepy is a library which makes it easy to use the Twitter API.
To install it, type:
pip install tweepy
Get API Keys
You will need to visit https://apps.twitter.com/ and follow Twitter's instructions for creating an app.
You will end up with a "Consumer Key" and a "Consumer Secret"
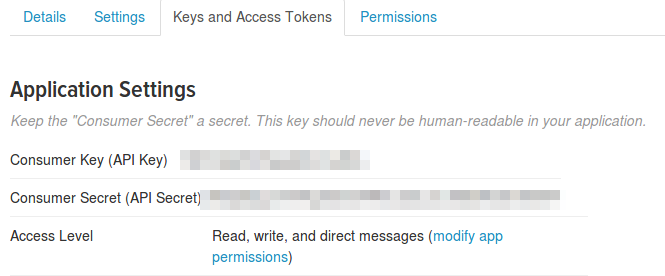
These are the secret tokens which belong to your app. What we need to do next is to sign in to the app and generate your personal tokens.
Get Tokens
- You can save this piece of code as
oauth.py
- You run it as
python oauth.py
import tweepy
consumer_key = "YOUR CONSUMER KEY HERE"
consumer_secret = "YOUR CONSUMER SECRET HERE"
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.secure = True
auth_url = auth.get_authorization_url()
print 'Visit this URL and authorise the app to use your Twitter account: ' + auth_url
verifier = raw_input('Type in the generated PIN: ').strip()
auth.get_access_token(verifier)
# Print out the information for the user
print "consumer_key = '%s'" % consumer_key
print "consumer_secret = '%s'" % consumer_secret
print "access_token = '%s'" % auth.access_token
print "access_token_secret = '%s'" % auth.access_token_secret
- It will print a URL, open that URL in your browser and it will take you to Twitter.
- Log in to Twitter with your account.
- Twitter will show you a PIN - 6 numbers long.
- Type the PIN into the Command Line.
- The program will display all your API Tokens.
So, how do we use them?
Using your Twitter tokens
- Add your tokens to this code.
- You can save this piece of code as
tweet.py
- You run it as
python tweet.py
- It will Tweet a message on your behalf.
import tweepy
# Consumer keys and access tokens, used for OAuth
consumer_key = 'YOUR CONSUMER KEY HERE'
consumer_secret = 'YOUR CONSUMER SECRET HERE'
access_token = 'YOUR ACCESS TOKEN HERE'
access_token_secret = 'YOUR ACCESS TOKEN SECRET HERE'
# OAuth process, using the keys and tokens
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
# Creation of the actual interface, using authentication
api = tweepy.API(auth)
# Send the tweet
message = 'This is a test'
api.update_status(message)
Get a list of all* the people you follow
- Add your tokens to this code.
- You can save this piece of code as
following.py
- You run it as
python following.py
- It will get the ID numbers of everyone* you follow and print them out.
import tweepy
# Consumer keys and access tokens, used for OAuth
consumer_key = 'YOUR CONSUMER KEY HERE'
consumer_secret = 'YOUR CONSUMER SECRET HERE'
access_token = 'YOUR ACCESS TOKEN HERE'
access_token_secret = 'YOUR ACCESS TOKEN SECRET HERE'
# OAuth process, using the keys and tokens
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
# Creation of the actual interface, using authentication
api = tweepy.API(auth)
# Get all the people the user follows
friends = api.friends_ids()
# Print out each one
for id in friends:
print(id)
* For technical reasons, this will only get the first 5,000 people you follow.
Let's save these to a file. Note the differences in this code and the previous piece of code.
import tweepy
import os
# Consumer keys and access tokens, used for OAuth
consumer_key = 'YOUR CONSUMER KEY HERE'
consumer_secret = 'YOUR CONSUMER SECRET HERE'
access_token = 'YOUR ACCESS TOKEN HERE'
access_token_secret = 'YOUR ACCESS TOKEN SECRET HERE'
# OAuth process, using the keys and tokens
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
# Creation of the actual interface, using authentication
api = tweepy.API(auth)
friends = sorted(api.friends_ids())
friendsFile = open("friends.txt","w")
for id in friends:
friendsFile.write(str(id) + "\n")
friendsFile.close()
You will now have a file called "friends.txt" which contains the ID Numbers of everyone you follow.
Handy for backing up your account :-)
One thought on “Easy Tutorial For Getting Twitter Friends Using Python & Tweepy”